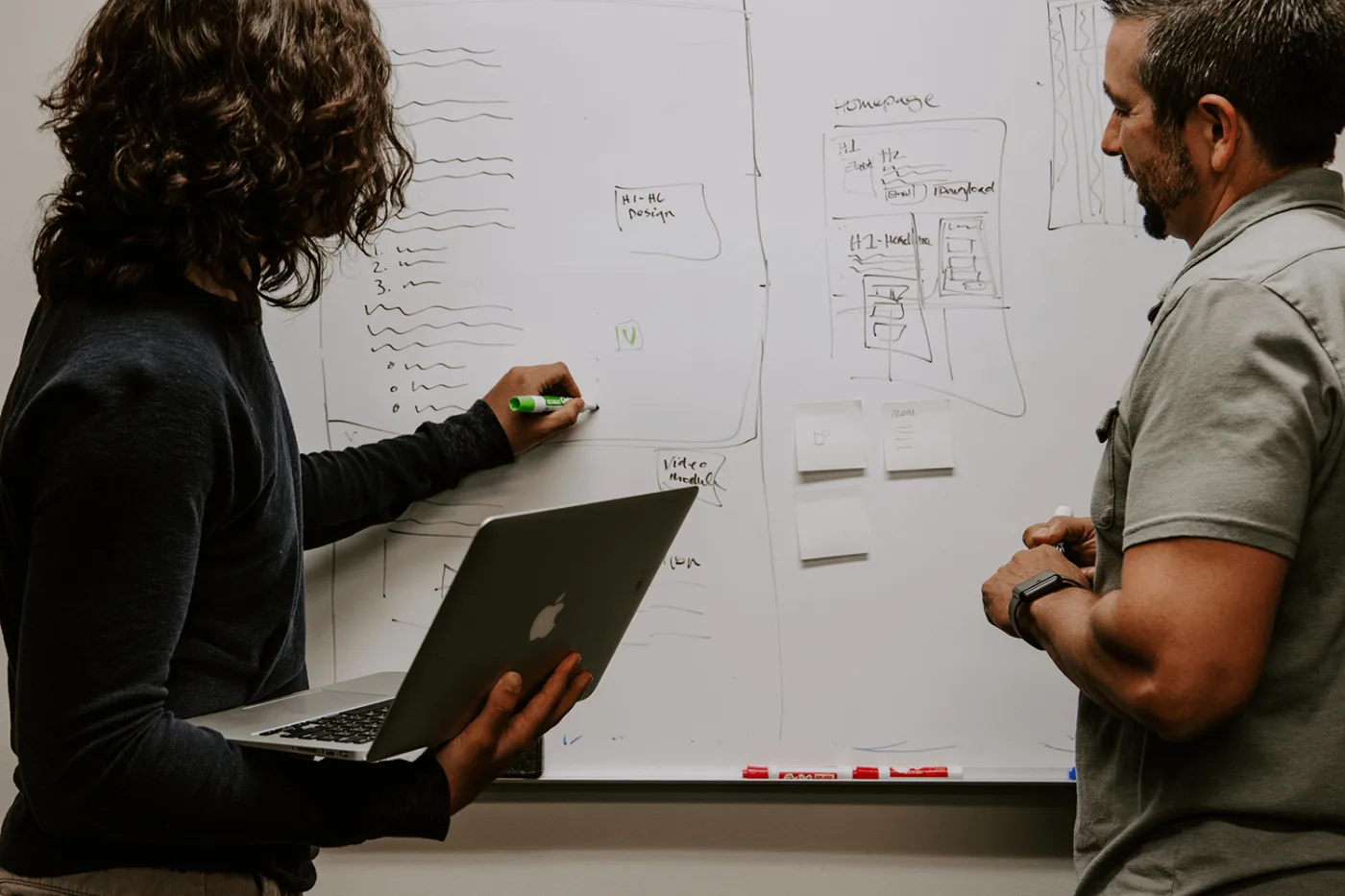
With the rapid advancements in web technologies, it can be overwhelming for aspiring developers to know where to begin and how to progress in their learning.
This is where a detailed learning roadmap can be invaluable. In this guide, I'll outline a comprehensive roadmap for frontend web developers, covering essential web technologies, CSS and JavaScript frameworks, web performance optimization, responsive web design, accessibility, version control, project and workflow management, and advanced CSS and JavaScript concepts. Whether you're a beginner or an experienced developer looking to expand your skill set, this roadmap will provide a structured approach to learning and help you reach your goals in frontend web development.
Essential Web Technologies
The foundation of frontend web development lies in mastering the essential web technologies that make up the building blocks of the web. These technologies include HTML, CSS, and JavaScript.
HTML
HTML (HyperText Markup Language) is used to structure and give meaning to the content of a webpage. It provides a standardized way of organizing and formatting text, images, videos, and other media. HTML is a markup language, meaning it uses tags and attributes to specify the structure and properties of different elements on a webpage.
Here's my suggested HTML learning path:
Basic HTML Syntax:
- Understanding HTML tags and attributes
- Basic HTML structure
- Creating HTML documents using text editors
HTML Document Structure:
- HTML5 document structure
- Creating HTML head and body sections
- Creating title, meta, and link elements
Text Markup:
- Using headings and paragraphs
- Creating lists (ordered and unordered)
- Styling text using emphasis, strong, and underline tags
Hyperlinks:
- Creating hyperlinks to external and internal pages
- Linking to email addresses and telephone numbers
- Using anchor tags to create named anchors
Images and Multimedia:
- Inserting images into HTML documents
- Adding alt and title attributes to images
- Embedding multimedia content using audio and video tags
Forms and Input:
- Creating forms using form tags
- Adding input fields (text, password, checkbox, radio, etc.)
- Using labels and placeholders
Tables:
- Creating tables using table, tr, th, and td tags
- Adding borders, padding, and spacing
- Merging cells and creating captions
Semantic HTML:
- Using semantic HTML tags (header, footer, nav, main, section, article, aside)
- Understanding the importance of semantic HTML for accessibility and SEO
Validation and Debugging:
- Validating HTML using W3C validators
- Debugging HTML using browser development tools
Advanced HTML Concepts:
- Creating responsive images using srcset and sizes attributes
- Using data attributes for custom data
- Creating HTML templates using the template tag
CSS
CSS (Cascading Style Sheets) is used to add style and design to HTML elements. It allows developers to control the layout, typography, colors, and other visual aspects of a webpage. CSS works by creating style rules that apply to specific HTML elements or groups of elements.
Here's my suggested CSS learning path:
Basic CSS Syntax:
- Understanding CSS rules and properties
- Creating CSS files and linking them to HTML documents
- Applying styles to HTML elements using selectors
Box Model:
- Understanding the box model (content, padding, border, margin)
- Applying styles to box model properties
- Box sizing and inheritance
Layout:
- Positioning elements using static, relative, absolute, and fixed positioning
- Floating elements and clearing floats
- Creating flexible layouts using display: flex and grid
Typography:
- Applying styles to text properties (font-family, font-size, line-height, etc.)
- Creating and using custom fonts with @font-face
- Adding special effects to text (text-shadow, text-decoration, etc.)
Colors and Backgrounds:
- Specifying colors using various color models (RGB, HEX, HSL)
- Applying color to text and backgrounds
- Using background images and gradients
Transitions and Animations:
- Creating smooth transitions between CSS properties
- Animating CSS properties using keyframes and animation properties
- Using CSS libraries for animations and transitions
Responsive Design:
- Using media queries to create responsive designs
- Creating fluid layouts and flexible images
- Mobile-first design approach
Preprocessors:
- Understanding CSS preprocessors (LESS, Sass, Stylus)
- Creating and using variables, mixins, and functions
- Compiling preprocessors to standard CSS
CSS Frameworks:
- Understanding CSS frameworks (Bootstrap, Foundation, Materialize)
- Using prebuilt styles and components
- Customizing framework styles and creating custom components
Debugging and Optimization:
- Using browser development tools for CSS debugging
- Optimizing CSS for performance (minification, combining, etc.)
- Understanding browser compatibility issues
JavaScript
JavaScript is a programming language that allows developers to create interactive and dynamic web content. It's used to add interactivity to web pages, such as animations, form validation, and event handling. JavaScript can also be used to create entire web applications, making it an essential skill for frontend web developers.
Here's my suggested JavaScript learning path:
Basic JavaScript Syntax:
- Understanding JavaScript variables and data types
- Creating and calling functions
- Using conditional statements and loops
Document Object Model (DOM):
- Understanding the DOM and how it relates to HTML
- Accessing and manipulating elements on a webpage using JavaScript
- Creating and removing HTML elements dynamically
Events:
- Understanding events and event listeners
- Responding to user interactions (click, hover, etc.)
- Creating custom events
ES6 and Beyond:
- Understanding new features in ECMAScript 6 and later versions
- Using arrow functions and template literals
- Destructuring objects and arrays
Asynchronous Programming:
- Understanding asynchronous programming and callbacks
- Using Promises and the async/await syntax
- Making asynchronous requests to APIs
JavaScript Frameworks:
- Understanding JavaScript frameworks (React, Vue.js, Angular)
- Creating and using components
- Understanding state and data binding
Data Structures and Algorithms:
- Understanding data structures (arrays, objects, maps, sets)
- Implementing sorting and searching algorithms
- Understanding time and space complexity
Testing:
- Understanding unit testing and test-driven development
- Using testing frameworks like Jest and Mocha
- Debugging JavaScript using browser development tools
Advanced JavaScript Concepts:
- Understanding functional programming concepts
- Using higher-order functions and closures
- Understanding the module pattern and code organization
In addition to these core technologies, it's important for frontend web developers to have a solid understanding of the Document Object Model (DOM). The DOM is a programming interface for web documents, and it allows developers to manipulate the content and structure of a webpage using JavaScript. Understanding the DOM is essential for creating dynamic and interactive web content.
Mastery of these essential web technologies is crucial for any frontend web developer, and they provide the foundation for all further learning in this field.
JavaScript Libraries and Frameworks
JavaScript libraries and frameworks are essential tools for any frontend web developer, as they provide pre-built solutions for common web development tasks and allow developers to work more efficiently. Here are some popular JavaScript libraries and frameworks used by frontend web developers:
React:
React is a JavaScript library for building user interfaces, developed by Facebook. It provides a declarative approach to building UI components, and allows for efficient updates through the use of a virtual DOM.
Vue.js:
Vue.js is a progressive JavaScript framework for building user interfaces. It emphasizes a component-based architecture, and provides simple and flexible API for creating reactive data bindings, animations, and transitions.
Angular:
Angular is a TypeScript-based JavaScript framework for building web applications. It provides a full-featured set of tools for building complex web applications, including a component-based architecture, reactive data bindings, and dependency injection.
Using JavaScript libraries and frameworks can greatly improve a developer's productivity and efficiency, allowing them to build complex web applications and user interfaces in less time. Understanding the strengths and weaknesses of each library and framework is important for selecting the right tools for each project.
Prototype Tools
Prototype tools are essential for frontend web developers who want to quickly create interactive prototypes of their designs without writing production-ready code. These tools allow developers to test and refine their designs before moving on to development, and can save significant time and resources. Here are some popular prototype tools used by frontend web developers:
Figma:
Figma is a web-based design and prototype tool that allows developers to create high-fidelity prototypes using a range of interactive components and animations. It also provides real-time collaboration features, making it easy to work with others remotely.
Adobe XD:
Adobe XD is a design and prototype tool that allows developers to create high-fidelity prototypes with interactive components and animations. It also provides integration with other Adobe products like Photoshop and Illustrator.
Sketch:
Sketch is a desktop-based design tool that allows developers to create high-fidelity designs and interactive prototypes. It also provides features for creating reusable design elements and symbols, making it easy to maintain consistency across designs.
Using prototype tools can greatly improve a developer's workflow and allow them to create and iterate on designs quickly and efficiently. Understanding the strengths and weaknesses of each tool is important for selecting the right tool for each project.
Web Performance Optimization
Web performance optimization is an essential aspect of frontend web development, as it directly impacts the user experience and can affect a website's search engine ranking. Here are some key concepts and techniques for optimizing web performance:
Page Speed:
Improving page speed is a crucial aspect of web performance optimization. This can be achieved through techniques such as minimizing file sizes, leveraging browser caching, and optimizing images and videos.
Code Optimization:
Writing optimized and efficient code is important for improving web performance. This can include techniques such as minification, compressing resources, and reducing the number of HTTP requests.
Content Delivery Network (CDN):
Using a CDN can greatly improve web performance by caching and delivering content from servers located closer to the user. This can reduce latency and improve loading times.
Responsive Design:
Designing for multiple devices and screen sizes can improve web performance by reducing the amount of data transferred and optimizing layout and rendering.
JavaScript Optimization:
Optimizing JavaScript can improve web performance by reducing the size of scripts and optimizing script execution. This can include techniques such as code splitting, lazy loading, and using web workers.
Performance Testing:
Testing and monitoring web performance is important for identifying areas of improvement and ensuring that optimizations are effective. This can include tools such as Google PageSpeed Insights and WebPageTest.
Optimizing web performance is an ongoing process that requires continuous monitoring and improvement. Understanding these key concepts and techniques can help frontend web developers create high-performing websites that deliver a seamless user experience.
Cross-Browser Compatibility
Cross-browser compatibility is a crucial aspect of frontend web development, as websites and applications need to be accessible and function consistently across different browsers and devices. Here are some key concepts and techniques for achieving cross-browser compatibility:
Feature Detection:
Feature detection involves checking whether a particular browser supports a specific feature or functionality before using it. This can help ensure that the website or application functions consistently across different browsers.
Vendor Prefixes:
Vendor prefixes are used to specify CSS properties that are not yet standardized and may be implemented differently across different browsers. Adding vendor prefixes ensures that the website or application displays correctly on different browsers.
Polyfills:
Polyfills are JavaScript code that fills in the gaps for browsers that do not support certain features. This can help ensure that the website or application functions consistently across different browsers.
Testing:
Testing the website or application across different browsers and devices is crucial for ensuring cross-browser compatibility. This can include using tools such as BrowserStack or running virtual machines to test on different operating systems.
Progressive Enhancement:
Progressive enhancement involves designing and building the website or application to function on all browsers, and then adding advanced features and functionalities for browsers that support them. This ensures that the website or application functions consistently across different browsers.
Achieving cross-browser compatibility is an ongoing process that requires continuous testing and improvement. Understanding these key concepts and techniques can help frontend web developers create websites and applications that function consistently and are accessible to all users.
Accessibility
Accessibility is an important aspect of frontend web development, as it ensures that websites and applications are accessible to all users, regardless of their abilities or disabilities. Here are some key concepts and techniques for achieving accessibility:
Semantic HTML:
Using semantic HTML, which is designed to convey meaning rather than just presentation, can improve accessibility by allowing screen readers and other assistive technologies to accurately interpret and convey information to users.
Alt Tags:
Alt tags are used to provide alternative text for images, which is important for users who are visually impaired and rely on assistive technologies to interpret the content of a webpage.
Keyboard Navigation:
Ensuring that websites and applications can be navigated using only a keyboard is crucial for users with mobility or motor impairments who are unable to use a mouse or touch screen.
Color Contrast:
Ensuring that text and other visual elements have sufficient contrast can improve accessibility for users with low vision or color blindness.
ARIA:
ARIA (Accessible Rich Internet Applications) is a set of attributes that can be added to HTML elements to provide additional information to assistive technologies, improving accessibility for users with disabilities.
Testing:
Testing the website or application with assistive technologies, such as screen readers or magnifiers, is important for ensuring accessibility. This can include using tools such as NVDA or JAWS.
Achieving accessibility is an ongoing process that requires continuous testing and improvement. Understanding these key concepts and techniques can help frontend web developers create websites and applications that are accessible to all users.
Version Control
In frontend web development, version control plays a vital role in tracking changes and enabling effective collaboration between developers. To make the most of version control, there are important concepts and techniques to consider. Here are some of the key ones to keep in mind:
Git:
Git is a popular version control system used by frontend web developers. It allows developers to track changes, collaborate on projects, and maintain different versions of a codebase.
Branching:
Branching allows developers to work on different versions of a codebase simultaneously. This can be useful for testing new features or making changes without affecting the main codebase.
Merging:
Merging involves combining different branches or versions of a codebase into a single codebase. This can be done once changes have been tested and approved.
Committing:
Committing involves saving changes to a codebase, along with a message describing the changes made. This helps developers track changes and identify issues.
Pull Requests:
Pull requests are a way for developers to request that their changes be reviewed and merged into the main codebase. This allows for collaboration and ensures that changes are thoroughly reviewed before being merged.
Hosting Platforms:
Hosting platforms, such as GitHub or GitLab, provide a platform for storing code repositories and collaborating with other developers.
Using version control is an essential aspect of frontend web development, and understanding these key concepts and techniques can help developers work effectively and collaboratively on projects.
Project and Workflow Management
Effective project and workflow management are vital components of frontend web development, as they help ensure timely and successful completion of projects. To achieve this, there are key concepts and techniques to consider. Below are some of the essential ones for managing projects and workflows in frontend web development:
Agile Methodology:
Agile methodology is a popular approach to project management that emphasizes flexibility and collaboration. It involves breaking down projects into small, manageable tasks and iterating on them in short sprints.
Project Management Tools:
Using project management tools, such as Trello or Asana, can help frontend web developers track tasks, deadlines, and progress.
Continuous Integration and Deployment:
Continuous integration and deployment (CI/CD) involves automating the testing, integration, and deployment of code changes. This can help ensure that changes are thoroughly tested and deployed quickly and efficiently.
Code Reviews:
Conducting code reviews can help ensure that code is high-quality, well-documented, and follows best practices. This can also help identify issues and improve overall code quality.
Collaborative Tools:
Using collaborative tools, such as Slack or Microsoft Teams, can help frontend web developers communicate effectively and share information quickly and easily.
Time Management:
Effective time management is crucial for frontend web developers, as it allows them to prioritize tasks, manage deadlines, and maintain a healthy work-life balance.
By understanding and implementing these key concepts and techniques for project and workflow management, frontend web developers can improve their efficiency and effectiveness, and ensure that projects are completed on time and to a high standard.
Conclusion
In conclusion, frontend web development is a constantly evolving field that requires a diverse skill set and a willingness to learn and adapt. By following the essential learning roadmap for frontend web development, including mastering essential web technologies, JavaScript, CSS, and other important concepts such as cross-browser compatibility, accessibility, version control, and project and workflow management, developers can build high-performing, user-friendly websites and applications. Ongoing learning and improvement are necessary to keep up with the latest trends and technologies in frontend web development, but by embracing these key concepts and techniques, frontend web developers can achieve success and make a significant impact in the industry.